It‘s very important for any website to have a support form, which we call a contact form or support form. Through the website contact form, any user can easily contact the website administrator by messaging. When the user enters the details in the contact form and sends, the details entered in the form are sent to the email id of the website administrator.
How to create contact form using PHP?
In this tutorial, we will create a contact form using PHP programming. The contact form is used to contact by user with site admin. Admin receives user details on email.
In this process, we do not need to create any MYSQL database. We will use PHP mail() function and form.
Let’s create a contact form in PHP –
First of all, we create an HTML form. The HTML form contains name, email , phone number and message . You can add more fields according to your needs.
HTML contact form -
<form action="" method="POST">
<label> Name :-</label>
<input type="text" name="name" class="form-control" required>
<label>Email :-</label>
<input type="email" name="email" class="form-control" required>
<label>Phone Number :-</label>
<input type="number" name="phone" class="form-control" required>
<label> Message :-</label>
<textarea name="msg" cols="10" rows="5" class="form-control" required></textarea>
<input type="submit" name="submit_form" value="Send" class="btn-primary">
</form>
In the HTML form above, we have made some fields like – name, email, phone number and message. The form will be used to receive information from the user. User will enter details and click on send button. The information entered by user will be delivered on admin email address.
Let’s create PHP scripts form contact form –
The PHP scripts will handle the logical part of contact form. We will handle the contact form values using post method. After handling information from the form, we will send the all details (name ,email ,phone number and message body ) on the email of website admin . You will able to setup any email id to receive all mails from the contact form page . In the contact form, we use mail() function . The mail() function is a inbuilt function of PHP , which is used to delivered mail by default host SMTP .
The mail() function is a best function for contact form .
Create PHP scripts for contact form –
<?php
if(isset($_POST['submit_form']))
{
$name=$_POST['name'];
$email=$_POST['email'];
$msg=$_POST['msg'];
$phone=$_POST['phone'];
$FromName="Techno Smarter";
$FromEmail="[email protected]";
$ReplyTo=$email;
$toemail="[email protected]";
$subject="Techno Smarter Contact form";
$message='Name:-'.$name.'<br>Email :-' .$email.'<br> Phone No:-'.$phone.'<br> Message:-'.$msg;
$headers = "MIME-Version: 1.0\n";
$headers .= "Content-type: text/html; charset=iso-8859-1\n";
$headers .= "From: ".$FromName." <".$FromEmail.">\n";
$headers .= "Reply-To: ".$ReplyTo."\n";
$headers .= "X-Sender: <".$FromEmail.">\n";
$headers .= "X-Mailer: PHP\n";
$headers .= "X-Priority: 1\n";
$headers .= "Return-Path: <".$FromEmail.">\n";
if(mail($toemail, $subject, $message, $headers,'-f'.$FromEmail) ){
$hide=1;
echo '<div class="success"><center><span style="font-size:100px;">✅</span></center> <br> Thank you <strong>'.$name.',</strong> Your message has been sent. We will reply soon as possible. </div> ';
} else {
echo "The server failed to send the message. Please try again later or Make sure , you are using live server for email.";
}
}
?>
In the code above, we have created many variables .
From Name – Website title.
From Email – Website official email like- [email protected]
To Email – Admin receiver email.
Subject – Set any text for subject .
Headers– These headers are compulsory for mail . You can remove content-type if you do not want to send HTML text.
The mail() function allows many parameters are below .
mail(to,subject,message,headers,parameters);
We have already discussed above .
If you are getting confused to create a file like contact.php.
You can use PHP scripts in the same contact file or create another file for those scripts.
contact.php
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1">
<title> Contact Form </title>
</head>
<body>
<div class="container">
<?php
if(isset($_POST['submit_form']))
{
$name=$_POST['name'];
$email=$_POST['email'];
$msg=$_POST['msg'];
$phone=$_POST['phone'];
$FromName="Techno Smarter";
$FromEmail="[email protected]";
$ReplyTo=$email;
$toemail="[email protected]";
$subject="Techno Smarter Contact form ";
$message='Name:-'.$name.'<br>Email :-' .$email.'<br> Phone No:-'.$phone.'<br> Message:-'.$msg;
$headers = "MIME-Version: 1.0\n";
$headers .= "Content-type: text/html; charset=iso-8859-1\n";
$headers .= "From: ".$FromName." <".$FromEmail.">\n";
$headers .= "Reply-To: ".$ReplyTo."\n";
$headers .= "X-Sender: <".$FromEmail.">\n";
$headers .= "X-Mailer: PHP\n";
$headers .= "X-Priority: 1\n";
$headers .= "Return-Path: <".$FromEmail.">\n";
if(mail($toemail, $subject, $message, $headers,'-f'.$FromEmail) ){
$hide=2;
echo '<div class="success"><center><span style="font-size:100px;">✅</span></center> <br> Thank you <strong>'.$name.',</strong> Your message has been sent. We will reply soon as possible. </div> ';
} else {
echo "The server failed to send the message. Please try again later or Make sure , you are using live server for email.";
}
}
?>
<?php if(!isset($hide)) { ?>
<h2>Contact Us ⛳</h2>
<form action="" method="POST">
<label> Name :-</label>
<input type="text" name="name" class="form-control" required>
<label>Email :-</label>
<input type="email" name="email" class="form-control" required>
<label>Phone Number :-</label>
<input type="number" name="phone" class="form-control" required>
<label> Message :-</label>
<textarea name="msg" cols="10" rows="5" class="form-control" required></textarea>
<input type="submit" name="submit_form" value="Send" class="btn-primary">
</form>
<?php }?>
</div>
</body>
</html>
We haven't designed the contact form yet.We will use CSS style to design contact form.
Use CSS to design contact form.
<style type="text/css">
body{
background-color: #f1f1f1;
}
.form-control {
width: 100%;
height: 34px;
padding: 6px 12px;
font-size: 14px;
color: #555;
background-color: #fff;
border: 1px solid #ccc;
border-radius: 4px;
}
.btn-primary {
padding: 6px 12px;
font-size: 14px;
font-weight: 400;
cursor: pointer;
border: 1px solid transparent;
border-radius: 4px;
background-color: #337ab7;
color: #fff;
}
textarea.form-control {
height: auto;
}
.container
{
margin-left: 30%;
width: 400px ;
margin-top: 6%;
background-color: #fff;
padding: 30px;
padding-right: 60px;
box-shadow: 10px 5px 5px grey;
}
label {
font-size: 18px;
}
.success
{
margin: 5px auto;
border-radius: 5px;
border: 3px solid #fff;
background: #33CC00;
color: #fff;
font-size: 20px;
padding: 10px;
box-shadow: 10px 5px 5px grey;
}
</style>
Now, you can test your contact form. If you are testing on local host without any SMTP then you will get an error. Kindly setup SMTP in local host or test on live server. Upload contact form file on liver server and test. You will get successful message on live server.
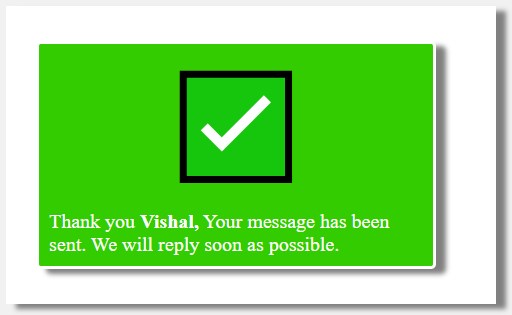
Live contact form on your website.
Recommended Posts:-