In the previous tutorial, we just discussed how to upload and display image on an HTML page using PHP without editing and deleting the image.
In this tutorial, you will learn how to upload the image, display, edit, and delete the image from the folder and database table using PHP and MYSQL database.
As know that PHP CRUD application is very important to create website pages using PHP but image CRUD application is small different from CRUD application with image.
How to upload image, display, edit, and delete using PHP and MYSQL?
The CRUD application with image helps to upload image, display images on HTML pages in the HTML table, and delete the image from the database but there are few differences if you work with the image file.
1. In the image file upload operation, we use enctype="multipart/form-data" to handle the image file.
2. We move the image to the "uploads" folder. We will need to create "uploads" folder to move all images to the same folder.
3. When we edit an image the old image should be removed (delete ) from the folder and move to the new image.
4. When we perform a delete operation in CRUD application with image, we delete the image from the folder and then delete the image from the database.
Let’s create a database –
Create a database table –
First of all, create a table in your database using the query below.
CREATE TABLE `items` (
`id` int(11) NOT NULL AUTO_INCREMENT,
`image` varchar(255) DEFAULT NULL,
`title` varchar(255) DEFAULT NULL,
PRIMARY KEY (`id`)
)
In the database table above, we created an items table. The image column will be used to insert the image name with the extension in the data table. The title column is an extra field for the image title or page title. This is just for testing.
Create a connection file –
This connection file will be used to connect the HTML page to the database using PHP.
Create a file config.php
config.php
<?php
$dbHost = 'localhost';
$dbName = 'tutedb';
$dbUsername = 'root';
$dbPassword = '';
$db= mysqli_connect($dbHost, $dbUsername, $dbPassword, $dbName);
?>
Kindly set the correct connection details.
Create a folder to save all images –
All images move to the folder on the server. We can store the image in the database but the best thing is to upload and move the image file to the folder.
Now, create a folder – uploads
Create this folder in the same path where you will create the PHP file. You can set a path after that.
Create PHP scripts to upload the image.
As know that the home page of any website is index.php. Now we will create index.php PHP.
index.php
<?php require_once("config.php");?>
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1">
<link rel="stylesheet" type="text/css" href="style.css">
<title>Upload image, display, edit and delete in PHP </title>
</head>
<body>
<div class="container_display">
<span style="float:right;"><a href="upload.php"><button class="btn-primary">Upload New image </button></a></span>
<br><br>
<?php
if(isset($_GET['image_success']))
{
echo '<div class="success">Image Uploaded successfully</div>';
}
if(isset($_GET['action']))
{
$action=$_GET['action'];
if($action=='saved')
{
echo '<div class="success">Saved </div>';
}
elseif($action=='deleted')
{
echo '<div class="success">Image Deleted Successfully ... </div>';
}
}
?>
<table cellpadding="10">
<tr>
<th> Image</th>
<th>Title</th>
<th>Action</th>
</tr>
<?php $res=mysqli_query($db,"SELECT* from items ORDER by id DESC");
while($row=mysqli_fetch_array($res))
{
echo '<tr>
<td><img src="uploads/'.$row['image'].'" height="200"></td>
<td>'.$row['title'].'</td>
<td><a href="edit.php?id='.$row['id'].'"><button class="btn-primary">Edit </button></a>
<br> <br>
<a href='delete.php?id='.$row['id'].'' onClick='return confirm("Are you sure you want to delete?")'"><button class="btn-primary btn_del">Delete</button></a>
</td>
</tr>';
} ?>
</table>
</div>
</body>
</html>
In the index file –
1. All images will be displayed on the index page.
2. We created an edit and delete button with table row id. The id will be used to edit the image and delete the image on another page.
3. We create an HTML table to display all images one by one using PHP and MYSQL database.
4. We created "upload new image" button to redirect to the upload page.User can upload the image from the upload page.
Let’s create an upload.php file that will help to upload the image.
Create a stylesheet file -
Create a file style.css to design.
style.css
body{
background-color: #f1f1f1;
}
.form-control {
width: 100%;
height: 25px;
padding: 6px 12px;
font-size: 14px;
color: #555;
background-color: #fff;
border: 1px solid #ccc;
border-radius: 4px;
}
.btn-primary {
padding: 6px 12px;
font-size: 14px;
font-weight: 400;
cursor: pointer;
border: 1px solid transparent;
border-radius: 4px;
background-color: #337ab7;
color: #fff;
}
.btn_del {
background-color: #FF5733 !important;
}
.container
{
margin-left: 30%;
width: 400px ;
background-color: #fff;
padding: 10px;
padding-right: 40px;
border: 1px solid #ccc;
border-radius: 4px;
}
.container_display
{
margin-left: 10%;
width: 900px ;
background-color: #fff;
padding: 10px;
padding-right: 40px;
border: 1px solid #ccc;
border-radius: 4px;
}
label {
font-size: 16px;
}
.success
{
margin: 5px auto;
border-radius: 5px;
border: 3px solid #fff;
background: #33CC00;
color: #fff;
font-size: 20px;
padding: 10px;
box-shadow: 10px 5px 5px grey;
}
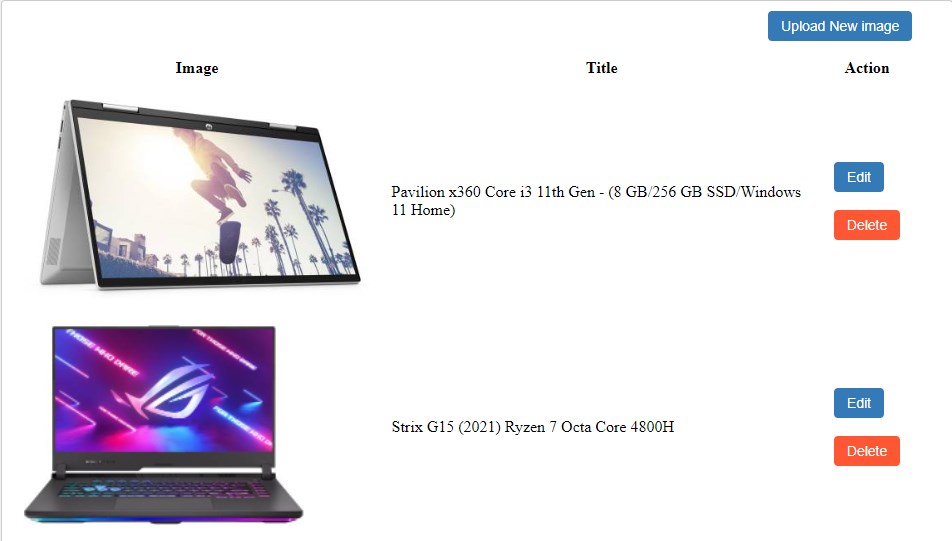
upload.php
<?php require_once("config.php");?>
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1">
<link rel="stylesheet" type="text/css" href="style.css">
<title>Image Upload in PHP and MYSQL database</title>
</head>
<body>
<?php
if(isset($_POST['form_submit']))
{
$title=$_POST['title'];
$folder = "uploads/";
$image_file=$_FILES['image']['name'];
$file = $_FILES['image']['tmp_name'];
$path = $folder . $image_file;
$target_file=$folder.basename($image_file);
$imageFileType=pathinfo($target_file,PATHINFO_EXTENSION);
//Allow only JPG, JPEG, PNG & GIF etc formats
if($imageFileType != "jpg" && $imageFileType != "png" && $imageFileType != "jpeg"
&& $imageFileType != "gif" ) {
$error[] = 'Sorry, only JPG, JPEG, PNG & GIF files are allowed';
}
//Set image upload size
if ($_FILES["image"]["size"] > 1048576) {
$error[] = 'Sorry, your image is too large. Upload less than 1 MB KB in size.';
}
if(!isset($error))
{
// move image in folder
move_uploaded_file($file,$target_file);
$result=mysqli_query($db,"INSERT INTO items(image,title) VALUES('$image_file','$title')");
if($result)
{
header("location:index.php?image_success=1");
}
else
{
echo 'Something went wrong';
}
}
}
if(isset($error)){
foreach ($error as $error) {
echo '<div class="message">'.$error.'</div><br>';
}
}
?>
<div class="container">
<form action="" method="POST" enctype="multipart/form-data">
<label>Image </label>
<input type="file" name="image" class="form-control" required >
<label>Title</label>
<input type="text" name="title" class="form-control">
<br><br>
<button name="form_submit" class="btn-primary"> Upload</button>
</form>
</div>
</body>
</html>
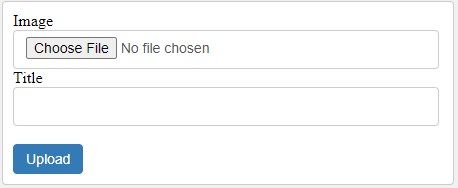
In the upload.php file above –
1. We created a form with an input type file. The image is also a file.
2. As you can see we used form attribute enctype="multipart/form-data" that is used to hold images from the form. Kindly do not forget to write it.
3. As you can see, we created an extra text box. This may be used as a page title or image title. If you don’t want it, you can remove it.
4. We created a PHP script to upload the image on a button click.
5. You can restrict the user to upload image size less than 1 MB or any other desired size in bytes.
if ($_FILES["image"]["size"] > 1048576) {
$error[] = 'Sorry, your image is too large. Upload less than 1 MB KB in size.';
}
1MB = 1048576 bytes
1. Restrict user to upload these file formats only .
if($imageFileType != "jpg" && $imageFileType != "png" && $imageFileType != "jpeg"
&& $imageFileType != "gif" ) {
$error[] = 'Sorry, only JPG, JPEG, PNG & GIF files are allowed';
}
2. We use the move_uploaded_file() function to move the image in the folder
3. After that, we created a query to insert the image name and title into the database table.
Image edit using PHP and MYSQL database –
The PHP edit operation is very important and small complex for beginners but you will understand it easily here. The update operation is done with the update query but in the image update operation, we delete the old image before updating the new image.
Let’s understand -
1. Fetch an old image from the database with row id.
2. Remove the image from the database when someone wants to update the new image on the button click
3. Move the new image to the same folder.
4. Update image name using update query in PHP.
This is the process, you will see in the edit operation.
Let’s create an edit.php file to update the image.
edit.php
<?php require_once("config.php"); $id=$_GET['id']; ?>
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1">
<link rel="stylesheet" type="text/css" href="style.css">
<title>Image Upload and edit in PHP and MYSQL database</title>
</head>
<body>
<?php
if(isset($_POST['update_submit']))
{
$title=$_POST['title'];
$folder = "uploads/";
$image_file=$_FILES['image']['name'];
$file = $_FILES['image']['tmp_name'];
$path = $folder . $image_file;
$target_file=$folder.basename($image_file);
$imageFileType=pathinfo($target_file,PATHINFO_EXTENSION);
if($file!=''){
//Set image upload size
if ($_FILES["image"]["size"] > 500000) {
$error[] = 'Sorry, your image is too large. Upload less than 500 KB in size.';
}
//Allow only JPG, JPEG, PNG & GIF
if($imageFileType != "jpg" && $imageFileType != "png" && $imageFileType != "jpeg"
&& $imageFileType != "gif" ) {
$error[] = 'Sorry, only JPG, JPEG, PNG & GIF files are allowed';
}
}
if(!isset($error))
{
if($file!='')
{
$res=mysqli_query($db,"SELECT* from items WHERE id=$id limit 1");
if($row=mysqli_fetch_array($res))
{
$deleteimage=$row['image'];
}
unlink($folder.$deleteimage);
move_uploaded_file($file,$target_file);
$result=mysqli_query($db,"UPDATE items SET image='$image_file',title='$title' WHERE id=$id");
}
else
{
$result=mysqli_query($db,"UPDATE items SET title='$title' WHERE id=$id");
}
if($result)
{
header("location:index.php?action=saved");
}
else
{
echo 'Something went wrong';
}
}
}
if(isset($error)){
foreach ($error as $error) {
echo '<div class="message">'.$error.'</div><br>';
}
}
$res=mysqli_query($db,"SELECT* from items WHERE id=$id limit 1");
if($row=mysqli_fetch_array($res))
{
$image=$row['image'];
$title=$row['title'];
}
?>
<div class="container" style="width:500px;">
<h1> Edit </h1>
<?php if(isset($update_sucess))
{
echo '<div class="success">Image Updated successfully</div>';
} ?>
<form action="" method="POST" enctype="multipart/form-data">
<label>Image Preview </label><br>
<img src="uploads/<?php echo $image;?>" height="100"><br>
<label>Change Image </label>
<input type="file" name="image" class="form-control">
<label>Title</label>
<input type="text" name="title" value="<?php echo $title;?>" class="form-control">
<br><br>
<button name="update_submit" class="btn-primary">Update </button>
</form>
</div>
</body>
</html>
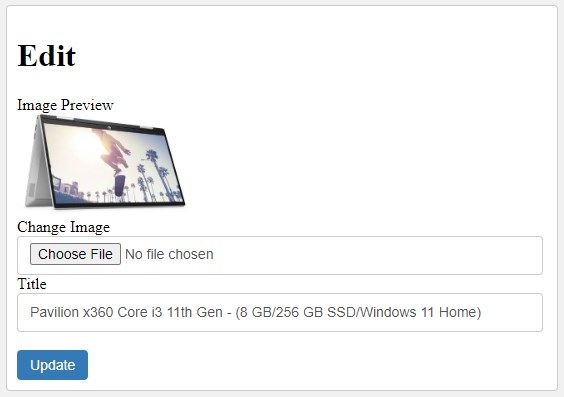
In the image update operation above –
1. First of all, we created a form to display the title and image from the database using PHP programming code.
2. We created a condition in the code that if someone browses an image from the computer then the old image should be removed and a new image should be moved into the folder and also update the image name in the database. Otherwise, if someone does not want to update then update only the title.
Delete image from the folder and database using PHP
The PHP delete operation is related to the update operation of the image file. In the delete operation, we remove the image from the folder like an update operation in PHP. After removing the image from the folder then we delete a row from the database using the delete query in PHP.
The delete operation is completed by the row id. We create an edit button with row id. Hold row id from the URL and use it at delete operation in PHP.
Let’s create a delete.php file.
delete.php
<?php require_once("config.php");
$id=$_GET['id'];
$res=mysqli_query($db,"SELECT* from items WHERE id=$id limit 1");
if($row=mysqli_fetch_array($res))
{
$deleteimage=$row['image'];
}
$folder="uploads/";
unlink($folder.$deleteimage);
$result=mysqli_query($db,"DELETE from items WHERE id=$id") ;
if($result)
{
header("location:index.php?action=deleted");
}
?>
In the image delete operation above –
1. We fetch the image name from the database and delete the image from the folder using the PHP unlink() function.
2. The PHP unlink() function is used to remove or delete images from the folder.
3. After that we created a delete query to delete the image from the database.
In this way, you can upload the image, display images, edit images and delete images using PHP and MYSQL database.
Recommended Posts:-